For a developer, creating a calendar in an app can be tricky. There are so many options for how to do it and the different libraries all have different advantages and disadvantages. jQuery datetimepicker is one of the newer libraries that has emerged as a front-runner for anyone looking to implement this functionality into their application.
The jQuery datetimepicker is an intuitive, user-friendly plugin that offers a date input widget for selecting dates. Using this plugin is a great way to offer your users an easy and efficient way of choosing a date.
This plugin is used to create an interactive JavaScript widget that lets users select a date on a calendar with their mouse or keyboard. The jQuery datetimepicker used Bootstrap 4 and can be customized in many ways, including background color, font size, and more.
Building & Publishing
You will need node.js to run this.
Update the version number.
Simple to Use jQuery Plugin to Animate SVG Pathspackage.json
bootstrap3/js/bootstrap-datetimepicker.js
bootstrap4/js/bootstrap-datetimepicker.js
Prepare:
npm install
How to build for Bootstrap 3:
npm run build3
How to build for Bootstrap 4:
How to Make a List in Pythonnpm run build4
Publish to the NPM registry:
npm publish
How to use:
Load the jQuery library, Bootstrap framework, and Font Awesome font.
Animated Donut Chart using JQuery and Snap.svg<!-- Font Awesome 5 Iconic Font -->
<link rel="stylesheet" href="/path/to/cdn/fontawesome.min.css" />
<!-- jQuery Library -->
<script src="/path/to/cdn/jquery.slim.min.js"></script>
<!-- Bootstrap Framework -->
<link rel="stylesheet" href="/path/to/cdn/bootstrap.min.css" />
<script src="/path/to/cdn/bootstrap.min.js"></script>
Load the following files into the document after downloading the package.
<!-- Stylesheet -->
<link rel="stylesheet" href="/path/to/css/bootstrap-datetimepicker.css" />
<!-- JavaScript -->
<script src="/path/to/js/bootstrap-datetimepicker.js"></script>
<!-- Languages -->
<script src="/path/to/locales/bootstrap-datetimepicker.fr.js"></script>
Use the input field to call the function.
<input type="text" value="2021-08-22 10:45" class="date" />
$(function(){
$(".date").datetimepicker();
});
You can customize the date and time picker.
$(".date").datetimepicker({
// parent container
container: 'body',
// language
// you can find all languages under the locales folder
// attribute: data-date-language
language: 'en',
// RTL mode
rtl: false,
// step size
// attributes: data-minute-step
minuteStep: 5,
// attributes: data-picker-position
pickerPosition: 'bottom-right',
// enable meridian views for day and hour views
// attributes: data-show-meridian
showMeridian: false,
// initial date
initialDate: new date(),
// z-index property
// attributes: data-z-index
zIndex: undefined,
// ISO-8601 valid datetime
format: 'mm/dd/yyyy',
// 0 = Sunday
// 6 = Saturday
// attributes: data-date-weekstart
weekStart: 0,
// start/end dates
// attributes: data-date-startdate and data-date-enddate
startDate: -Infinity,
endDate: Infinity,
// days of the week that should be disabled
// 0 = Sunday
// 6 = Saturday
// attributes: data-date-days-of-week-disabled
daysOfWeekDisabled: [],
// auto close the picker after selection
// attributes: data-date-autoclose
autoclose: false,
// auto close the picker after selection
// attributes: data-date-autoclose
autoclose: false,
// 0 or 'hour' for the hour view
// 1 or 'day' for the day view
// 2 or 'month' for month view (the default)
// 3 or 'year' for the 12-month overview
// 4 or 'decade' for the 10-year overview.
// attributes: data-start-view
startView: 2
// attributes:data-min-view
minView: 0,
// attributes: data-max-view
maxView: 4,
// select the view from which the date will be selected
// 'decade', 'year', 'month', 'day', 'hour'
// attributes: data-view-select
viewSelect: 0,
// show Today button
// attributes: data-date-today-btn
todayBtn: false,
// highlight the current date
todayHighlight: false,
// enable keyboard navigation
// attributes: data-date-keyboard-navigation
keyboardNavigation: true,
// whether or not to force parsing of the input value when the picker is closed
// attributes: data-date-force-parse
forceParse: false,
});
Methods for using APIs.
// show the datetime picker
$('#mydate').datetimepicker('show');
// hide the datetime picker
$('#mydate').datetimepicker('hide');
// update the datetime picker
$('#mydate').datetimepicker('update');
// destroy the datetime picker
$('#mydate').datetimepicker('remove');
// set start/end dates
$('#mydate').datetimepicker('setStartDate', '2020-01-01');
$('#mydate').datetimepicker('setEndDate', '2030-01-01');
// set the days of week that should be disabled
$('#date').datetimepicker('setDaysOfWeekDisabled', [0,6]);
The events
$('#mydate').datetimepicker()
.on('changeDate', function(ev){
// do something
})
.on('changeMonth', function(ev){
// do something
})
.on('changeYear', function(ev){
// do something
})
.on('outOfRange', function(ev){
// do something
})
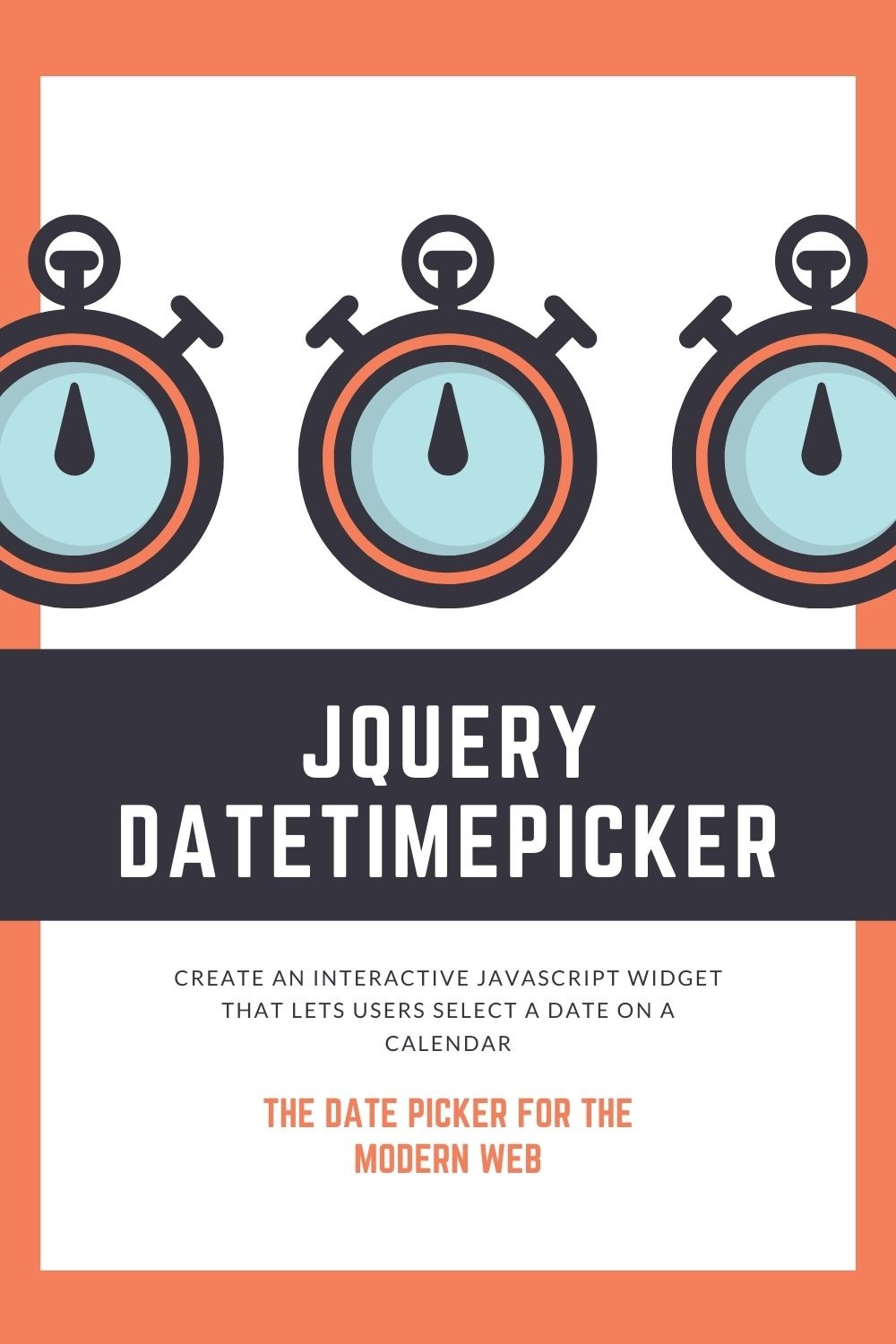