This is a JavaScript library protect Email address on website from spam. It uses dissimilar mystification procedures to hide the real email address and mailto
links along with the correct presentation methods to the user.
The hoax is to use simple ROT programming function (like Caesar cipher) to hide an email address and decode it every time the user clicks on a link. Because of the usage of the mousedown
event, any mouse button click is supposed to be calculated.
There are some additional obfuscation techniques as well that are used to protect email addresses as CSS converse technique, hidden <span> addition, and HTML comments insertion.
Prerequisites
There are no prerequisites for the library to use other than JavaScript permitted in a browser. The library is assembled with Babel transcompiler with default parameters of @babel/preset-env
preset. So, it should run without problems on any modern web browser. It was tried on:
- Mozilla Firefox v79
- Opera v67
- Google Chrome v80
The library was minified using Terser Plugin in webpack with default parameters. Source map was contained within the manufacture build. Webpack configuration files attachment can be found to the to the source root folder.
Add Product Tour with Hopscotch FrameworkInstallation
To use the library with your HTML documents, take in the email-protector.js
file (build
directory) using <script>
tag. You can download it and include as a local file. Future changes to the project won’t break your webpage:
<script src="assets/js/email-protector.js"></script>
Or library can be attached with the global link also:
<script src="https://raw.githubusercontent.com/bearbyt3z/email-protector.js/master/build/email-protector.js"></script>
Here is an example using default value of code parameter (which is 13):
20 Best CSS Grid Systems and Frameworksconsole.log(EmailProtector.rot('[email protected]'));
The expected output is:
[email protected]
Another example for ROT23 encoding (code 23):
console.log(EmailProtector.rot('[email protected]', 23));
The output will be:
5 Best Random Color Generator for Web Designers[email protected]
Inserting email address into HTML document
There are two methods available to pullout your email address link into HTML document.
Using append()
method
The first one assumes creating a HTML element with an arbitrary ID and calling append()
function with the ID and the encoded email address as parameters:
<body>
<!-- script with append() function can be placed anywhere in a HTML document -->
<script>
EmailProtector.append('email-protector', '[email protected]');
</script>
...
<p>
Please contact me at <span id="email-protector"></span>
</p>
</body>
Remember that the mailto link will be added as the last child of the provided HTML element. This is comparable behavior as in case of Node.appendChild() method which is called by the append() method.
25 Useful jQuery PluginsThis function uses window.onload event to add the email link to the specified HTML element, so it can be performed everyplace in a HTML document.
The above code will generate the following result:
<p>
Please contact me at
<span id="email-protector">
<a id="_k905fu05ixbj1tazna" href="znvygb:[email protected]">
<!-- mailto:[email protected] -->
ten
<!-- pre . -->
.
<!-- post . -->
niamod
<span>[email protected]</span>
<!-- pre @ -->
@
<!-- post @ -->
resu
</a>
</span>
</p>
Using write()
method
Second technique uses document.write()
method to print the mailto
link just after the <script>
tag, where the EmailProtector.write()
method was called:
<body>
<p>
Please contact me at
<script>
EmailProtector.write('[email protected]');
</script>
</p>
</body>
Notice that there is no parameter corresponding to the ID of a DOM element because the email link is placed where the <script>
tag arises.
The above code will generate a very similar result to the previous method:
<p>
Please contact me at
<script>
EmailProtector.write('[email protected]');
</script>
<a id="_k905fu05ixbj1tazna" href="znvygb:[email protected]">
<!-- mailto:[email protected] -->
ten
<!-- pre . -->
.
<!-- post . -->
niamod
<span>[email protected]</span>
<!-- pre @ -->
@
<!-- post @ -->
resu
</a>
</p>
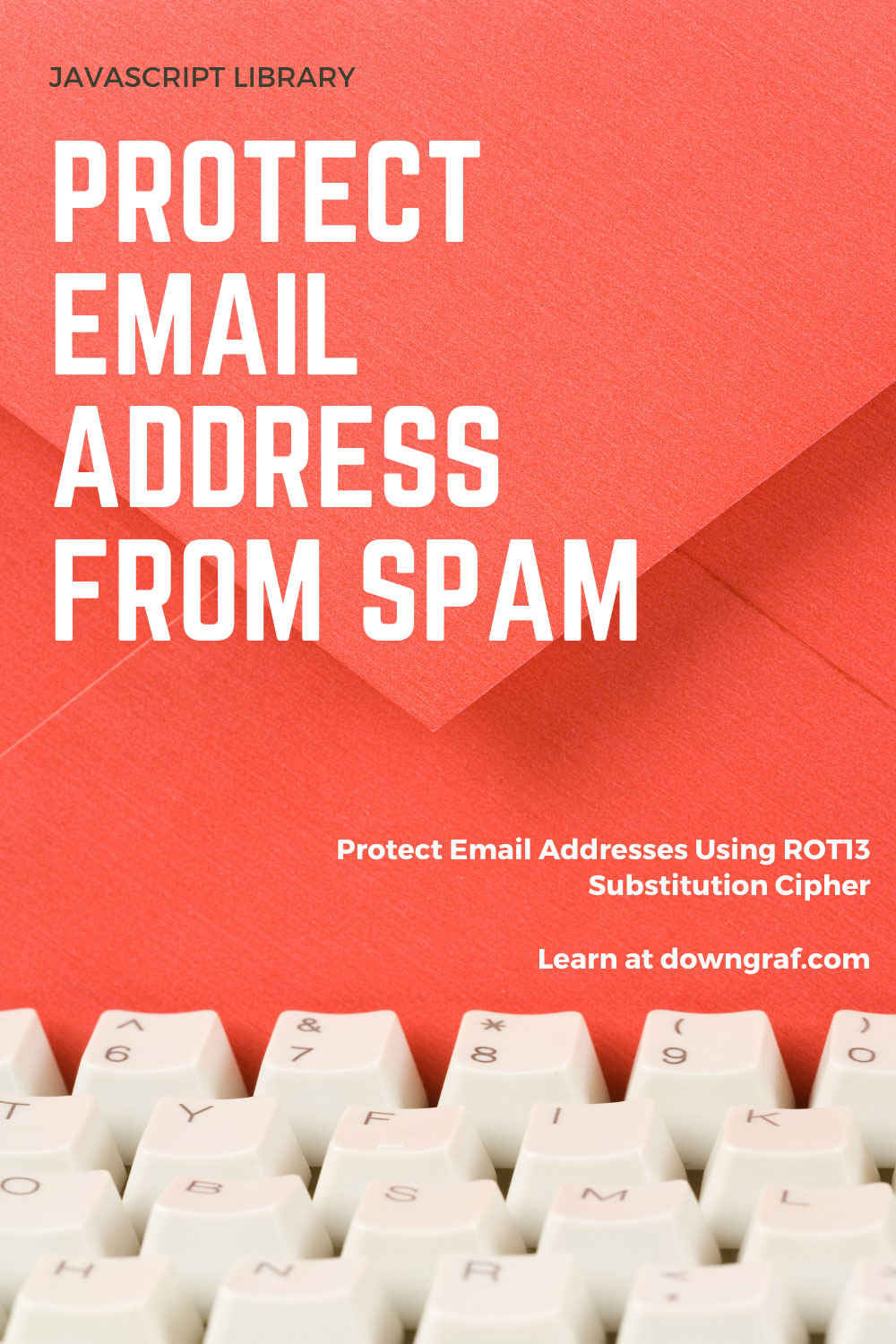
Email parameters
mailto
link parameters can be specified using first (in case of write()
) or second (in case of aplly()
) argument when calling each method. The argument must be a string in place of encoded email address or an object with any supported link parameters:
email
– an ROT encoded email address;subject
– the subject of an email message (should not be encoded);body
– the body of an email (not encoded);cc
– carbon copy of an email (also ROT encoded);bcc
– blind carbon copy (also ROT encoded).
Here is an example of all the above mentioned mailto
link parameters passed to the write()
function:
EmailProtector.write({
email: '[email protected]',
subject: 'Message from www',
body: 'Hi, I\'m contacting you',
cc: '[email protected]',
bcc: '[email protected]'
});
The cc
and bcc
parameters in the example correspond to [email protected]
and [email protected]
addresses respectively. As stated above they also have to be ROT encoded, because they will be subjected to the decoding process in the same way the main email address is (we don’t want to expose them to spam bots either).
cssReverse
Use the CSS reverse obfuscation technique to hide the real email address displayed as the mailto
link label. The email address is written in reverse order and the following CSS code is used to display it right to a user:
#some-unique-id {
unicode-bidi: bidi-override;
direction: rtl;
}
The default value is true
.
This option is taken into account only when linkLabel
is unset (undefined).
htmlComments
Adds another obfuscation technique using HTML comments. Dot (.
) and at (@
) signs are extended with comments in the following manner:
<!-- pre @ -->@<!-- post @ -->
Furthermore, there is another comment inserted at the beginning of the mailto
link label with some fake (generated) email address, e.g.:
<!-- mailto:[email protected] -->
The default value of this parameter is true
.
This option is taken into account only when linkLabel
is unset (undefined).
Using global parameters and configuration
Email message parameters for the library as well as configuration can be set as global using setGlobalParams()
and setGlobalConfig()
methods respectively.
<head>
...
<script>
EmailProtector.setGlobalParams({ email: '[email protected]', subject: 'Message from www' });
EmailProtector.setGlobalConfig({ code: 15, hiddenSpan: false, htmlComments: false });
</script>
</head>
<body>
<p>
Please contact me at
<script>
EmailProtector.write(); // calling without the email parameter
</script>
</p>
...
<p>
My email address:
<script>
EmailProtector.write();
</script>
</p>
...
</body>
To reset global parameters use resetParams()
method and to reset global configuration use resetConfig()
method:
EmailProtector.resetParams();
EmailProtector.resetConfig();
JavaScript input:
EmailProtector.write('[email protected]', {
linkLabel: 'Click here to contact me'
});
HTML result:
<a id="_k905fu05ixbj1tazna" href="znvygb:[email protected]">
Click here to contact me
</a>
JavaScript input:
EmailProtector.write('[email protected]', {
cssReverse: false,
hiddenSpan: false,
htmlComments: true
});
or simply:
EmailProtector.write('[email protected]');
HTML result:
<a id="_k905fu05ixbj1tazna" href="znvygb:[email protected]">
<!-- mailto:[email protected] -->
ten
<!-- pre . -->
.
<!-- post . -->
niamod
<span>[email protected]</span>
<!-- pre @ -->
@
<!-- post @ -->
resu
</a>
File Information
File Size | 117 KB |
Last Update | 1 Months Ago |
Date Published | 5 Months Ago |
License | GPL-3.0 License |